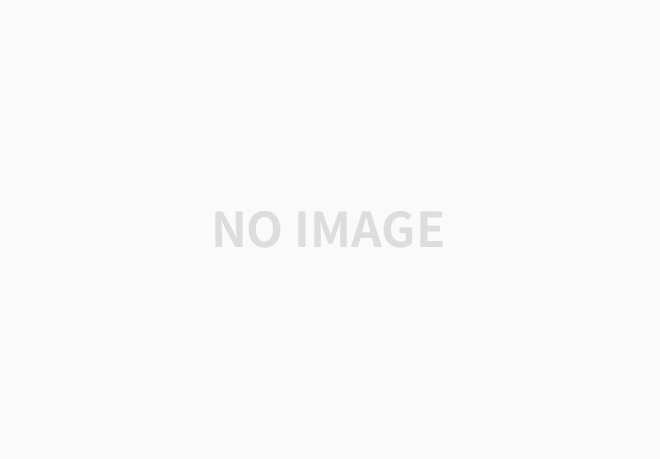
1: 서울
2: 대구
3: 대전
4: 부산
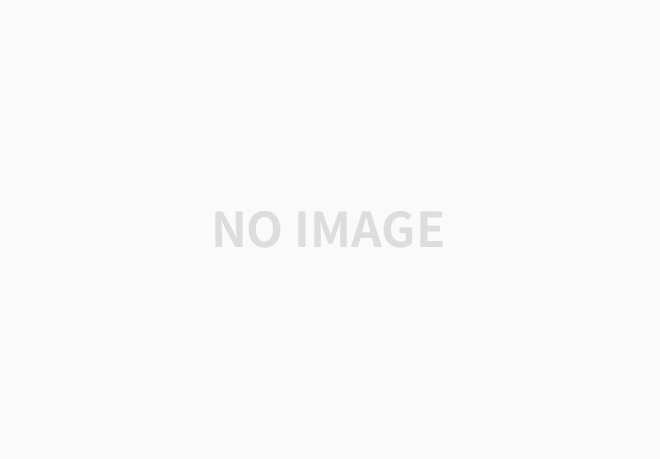
가중치 x
using System;
using System.Linq;
namespace Graph
{
class Node
{
public string data;
public Node(string data)
{
this.data = data;
}
}
class Graph
{
private Node[] nodes;
int[,] maps;
public Graph(int n)
{
this.nodes = new Node[n];
maps = new int[n+1, n+1];
}
public void AddVertex(Node node)
{
this.nodes.Append(node);
}
public void AddEdge(int n0, int n1)
{
maps[n0, n1] = 1;
maps[n1, n0] = 1;
}
public void Print()
{
for(int i = 0; i <= nodes.Length; i++)
{
for(int j = 0; j <= nodes.Length; j++)
{
Console.Write("{0} ", maps[i, j]);
}
Console.WriteLine();
}
}
}
}
using System;
using System.IO;
namespace Graph
{
class App
{
public App()
{
StreamReader sr = new StreamReader(Console.OpenStandardInput());
int n = int.Parse(sr.ReadLine());
string[] vertexs = sr.ReadLine().Split(' ');
Graph graph = new Graph(n);
Node node1 = new Node(vertexs[0]);
Node node2 = new Node(vertexs[1]);
Node node3 = new Node(vertexs[2]);
Node node4 = new Node(vertexs[3]);
graph.AddVertex(node1);
graph.AddVertex(node2);
graph.AddVertex(node3);
graph.AddVertex(node4);
for (int i = 0; i < n; i++)
{
string[] edge = sr.ReadLine().Split(' ');
int n0 = int.Parse(edge[0]);
int n1 = int.Parse(edge[1]);
graph.AddEdge(n0, n1);
}
graph.Print();
sr.Close();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Graph
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
가중치o
(AddEdge에 가중치 값만 추가)
using System;
using System.Linq;
namespace Graph
{
class Node
{
public string data;
public Node(string data)
{
this.data = data;
}
}
class Graph
{
private Node[] nodes;
int[,] maps;
public Graph(int n)
{
this.nodes = new Node[n];
maps = new int[n+1, n+1];
}
public void AddVertex(Node node)
{
this.nodes.Append(node);
}
public void AddEdge(int n0, int n1, int weight)
{
maps[n0, n1] = weight;
maps[n1, n0] = weight;
}
public void Print()
{
for(int i = 0; i <= nodes.Length; i++)
{
for(int j = 0; j <= nodes.Length; j++)
{
Console.Write("{0} ", maps[i, j]);
}
Console.WriteLine();
}
}
}
}
using System;
using System.IO;
namespace Graph
{
class App
{
public App()
{
StreamReader sr = new StreamReader(Console.OpenStandardInput());
int n = int.Parse(sr.ReadLine());
string[] vertexs = sr.ReadLine().Split(' ');
Graph graph = new Graph(n);
Node node1 = new Node(vertexs[0]);
Node node2 = new Node(vertexs[1]);
Node node3 = new Node(vertexs[2]);
Node node4 = new Node(vertexs[3]);
graph.AddVertex(node1);
graph.AddVertex(node2);
graph.AddVertex(node3);
graph.AddVertex(node4);
for (int i = 0; i < n; i++)
{
string[] edge = sr.ReadLine().Split(' ');
int n0 = int.Parse(edge[0]);
int n1 = int.Parse(edge[1]);
int weight = int.Parse(edge[2]);
graph.AddEdge(n0, n1, weight);
}
graph.Print();
sr.Close();
}
}
}

'알고리즘 > 수업 과제' 카테고리의 다른 글
[알고리즘] 재귀함수 (1) | 2023.02.08 |
---|