*BRAWLSTARS*
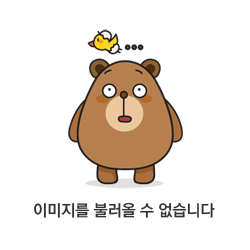
[브롤러]
---------
[속성]
---------
[기능]
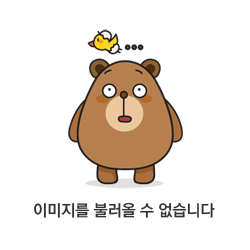
TICK[장거리딜러]
----------
[속성]
공격력 3*640
HP 2200
이동속도 2.4
----------
[기능]
공격하기
사망
이동하기
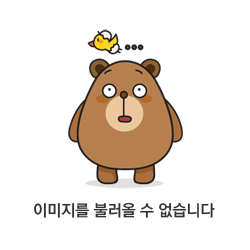
CHESTER [장거리 딜러]
----------
[속성]
공격력 720
HP 3300
이동속도 2.57
----------
[기능]
공격하기_1번째 1개 구체, 2번째 2, 3번째 3까지
사망
이동하기
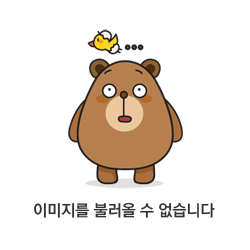
EMZ [중거리 딜러]
----------
[속성]
공격력 520
HP 3600
이동속도 2.4
----------
[기능]
공격하기_spray
사망
이동하기
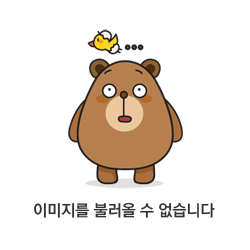
POCO[서포터]
----------
[속성]
공격력 760
회복량 700
HP 4000
이동속도 2.4
----------
[기능]
공격하기
사망
이동하기
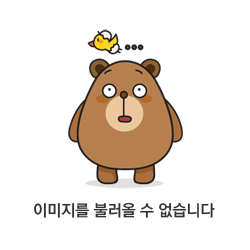
SPROUT [중거리 딜러]
----------
[속성]
공격력 980
HP 3000
이동속도 2.4
----------
[기능]
공격하기
사망
이동하기
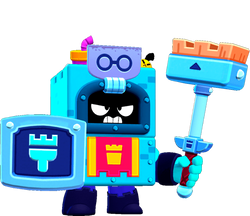
ASH[탱커]
----------
[속성]
공격력 800
HP 5400
이동속도 2.4
----------
[기능]
공격하기
사망
이동하기
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BRAWLSTARS
{
class Program
{
static void Main(string[] args)
{
APP app = new APP();
}
}
}
APP
using System;
namespace BRAWLSTARS
{
class APP
{
//생성자
public APP()
{
Console.WriteLine("APP 생성자");
TICK tick = new TICK();
Console.WriteLine(tick.damage);
Console.WriteLine(tick.hp);
Console.WriteLine(tick.speed);
tick.Move();
tick.Attack();
tick.Die();
Console.WriteLine();
CHESTER chester = new CHESTER();
Console.WriteLine(chester.damage);
Console.WriteLine(chester.hp);
Console.WriteLine(chester.speed);
chester.Move();
chester.Attack();
chester.Attack();
chester.Attack();
chester.Attack();
chester.Die();
Console.WriteLine();
EMZ emz = new EMZ();
Console.WriteLine(emz.damage);
Console.WriteLine(emz.hp);
Console.WriteLine(emz.speed);
emz.Move();
emz.Attack();
emz.Die();
Console.WriteLine();
POCO poco = new POCO();
Console.WriteLine(poco.damage);
Console.WriteLine(poco.heal);
Console.WriteLine(poco.hp);
Console.WriteLine(poco.speed);
poco.Move();
poco.Attack();
poco.Heal();
poco.Die();
Console.WriteLine();
SPROUT sprout = new SPROUT();
Console.WriteLine(sprout.damage);
Console.WriteLine(sprout.hp);
Console.WriteLine(sprout.speed);
sprout.Move();
sprout.Attack();
sprout.Die();
Console.WriteLine();
ASH ash = new ASH();
Console.WriteLine(ash.damage);
Console.WriteLine(ash.hp);
Console.WriteLine(ash.speed);
ash.Move();
ash.Attack();
ash.Die();
Console.WriteLine();
}
}
}
TICK
using System;
namespace BRAWLSTARS
{
class TICK
{
public int damage;
public int hp;
public float speed;
//생성자
public TICK()
{
Console.WriteLine("TICK 생성자");
this.damage = 640;
this.hp = 2200;
this.speed = 2.4f;
}
public void Attack()
{
Console.WriteLine("지뢰를 던졌습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
}
}
CHESTER
using System;
namespace BRAWLSTARS
{
class CHESTER
{
static int MAX_COUNT_BALL = 3;
public int nowBall;
public int damage;
public int hp;
public float speed;
//생성자
public CHESTER()
{
Console.WriteLine("CHESTER 생성자");
this.nowBall = 1;
this.damage = 720;
this.hp = 3300;
this.speed = 2.57f;
}
public void Attack()
{
this.damage = 720 * this.nowBall;
Console.WriteLine("{0}개의 구체로 공격하였습니다.데미지는 {1}", nowBall, damage);
this.nowBall++;
if (this.nowBall > MAX_COUNT_BALL)
{
this.nowBall = 1;
}
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
}
}
EMZ
using System;
namespace BRAWLSTARS
{
class EMZ
{
public int damage;
public int hp;
public float speed;
//생성자
public EMZ()
{
Console.WriteLine("EMZ 생성자");
this.damage = 520;
this.hp = 3600;
this.speed = 2.4f;
}
public void Attack()
{
Console.WriteLine("공격하였습니다.");
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
}
}
POCO
using System;
namespace BRAWLSTARS
{
class POCO
{
public int damage;
public int hp;
public int heal;
public float speed;
//생성자
public POCO()
{
Console.WriteLine("POCO 생성자");
this.damage = 760;
this.hp = 4000;
this.heal = 700;
this.speed = 2.4f;
}
public void Attack()
{
Console.WriteLine("공격하였습니다.");
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
public void Heal()
{
Console.WriteLine("회복시켰습니다.");
}
}
}
SPROUT
using System;
namespace BRAWLSTARS
{
class SPROUT
{
public int damage;
public int hp;
public float speed;
//생성자
public SPROUT()
{
Console.WriteLine("SPROUT 생성자");
this.damage = 980;
this.hp = 3000;
this.speed = 2.4f;
}
public void Attack()
{
Console.WriteLine("공격하였습니다.");
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
}
}
ASH
using System;
namespace BRAWLSTARS
{
class ASH
{
public int damage;
public int hp;
public float speed;
//생성자
public ASH()
{
Console.WriteLine("ASH 생성자");
this.damage = 800;
this.hp = 5400;
this.speed = 2.4f;
}
public void Attack()
{
Console.WriteLine("공격하였습니다.");
}
public void Move()
{
Console.WriteLine("이동하였습니다.");
}
public void Die()
{
Console.WriteLine("죽었습니다.");
}
}
}
실행 결과
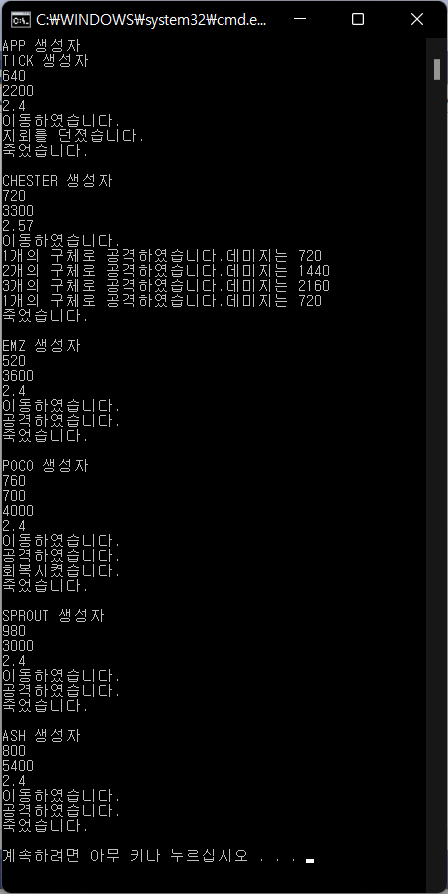
'C# > 수업과제' 카테고리의 다른 글
[ C# 10일차 ] 배열 직렬화/역직렬화 연습 (1) | 2023.01.13 |
---|---|
[ C# 10일차 ] 객체1 직렬화/역직렬화 연습 (1) | 2023.01.13 |
[ C# 8일차] List<T>를 이용한 Inventory 과제 (0) | 2023.01.11 |
[C# 7일차] 배열 Inventory 과제 (0) | 2023.01.10 |
[C# 5일차] Class 응용 과제&Method 활용_BRAWLSTARS(+궁극기, 스킬) (0) | 2023.01.06 |